PL/pgSQL – Variables
In PostgreSQL’s PL/pgSQL language, you can declare and use variables for storing and manipulating data within stored procedures, functions, and triggers. PL/pgSQL supports various data types for variables, including the standard SQL data types and custom types defined in your database.
Here’s how you can declare and use variables in PL/pgSQL:
Declaration:
You can declare a variable using the DECLARE
statement within the body of your PL/pgSQL code block. You should specify the variable name, data type, and optionally an initial value.
DECLARE variable_name data_type [ := initial_value ];
Initialization:
You can initialize a variable when you declare it, or you can set its value later using the :=
operator.
DECLARE my_var INT := 42; my_var := 100;
Usage:
You can use variables in your PL/pgSQL code like any other SQL expression.
DECLARE total_sales DECIMAL(10, 2) := 0;
SELECT SUM(sale_amount) INTO total_sales FROM sales;
Here’s a simple PL/pgSQL function that demonstrates variable usage:
CREATE OR REPLACE FUNCTION calculate_total(a INT, b INT) RETURNS INT
AS $$
DECLARE
result INT;
BEGIN
result := a + b;
RETURN result;
END;
$$ LANGUAGE plpgsql;
In this example:
- We declare a variable
result
of typeINT
. - We assign the sum of
a
andb
to theresult
variable. - We return the value of the
result
variable.
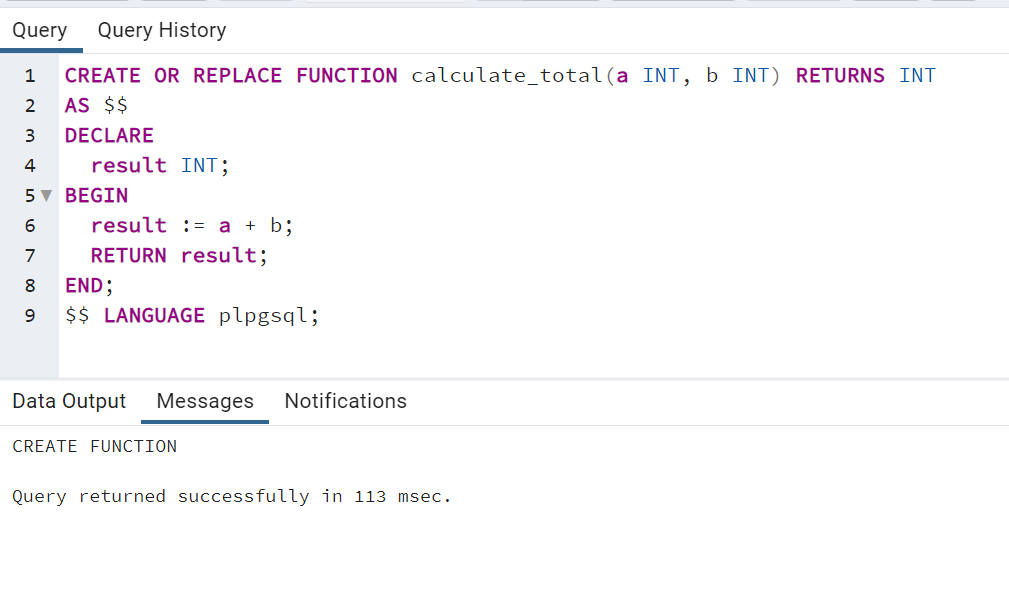
You can call this function like any other SQL function, passing values for a
and b
, and it will return the sum of the two values.
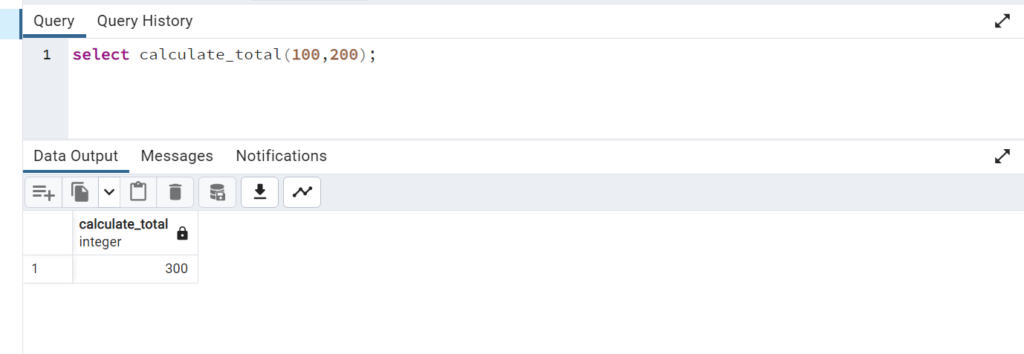