PostgreSQL – PROCEDURES
A procedure is a named block of code that performs a specific task. Procedures in PostgreSQL database are typically written in PL/pgSQL, which is a procedural language specifically designed for database programming. Procedures can accept parameters, perform actions, and return values.
Creating a Procedure:You can create a procedure using the CREATE OR REPLACE PROCEDURE
statement. The procedure can have input parameters (if needed) and a return type (if it’s expected to return a value).
CREATE OR REPLACE PROCEDURE procedure_name(parameter1 datatype, parameter2 datatype) LANGUAGE plpgsql AS $$ DECLARE -- Declare variables (if needed) BEGIN -- Procedure logic here END; $$
Defining the Procedure Logic:Inside the procedure, you can include PL/pgSQL statements to perform the desired actions. This can include SQL queries, control structures (such as IF
statements and loops), and variable assignments.
Calling the Procedure:You can call the procedure like any other function or stored procedure in PostgreSQL.
CALL procedure_name(parameter1_value, parameter2_value);
Here’s an example of a simple procedure that calculates the sum of two numbers and returns the result:
CREATE OR REPLACE PROCEDURE calculate_sum(a INT, b INT) LANGUAGE plpgsql AS $$ DECLARE result INT; BEGIN result := a + b; RAISE NOTICE 'Result value is: %', result; END; $$
You can call this procedure like this:
CALL calculate_sum(5,7); --Returns 12
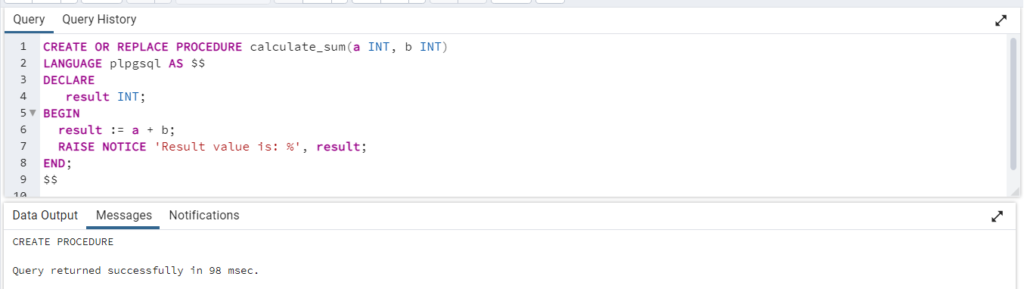

Procedures in PostgreSQL are useful for encapsulating complex logic, improving code organization, and reusing code across different parts of your application. They are commonly used for tasks such as data transformation, validation, and automation of database operations.